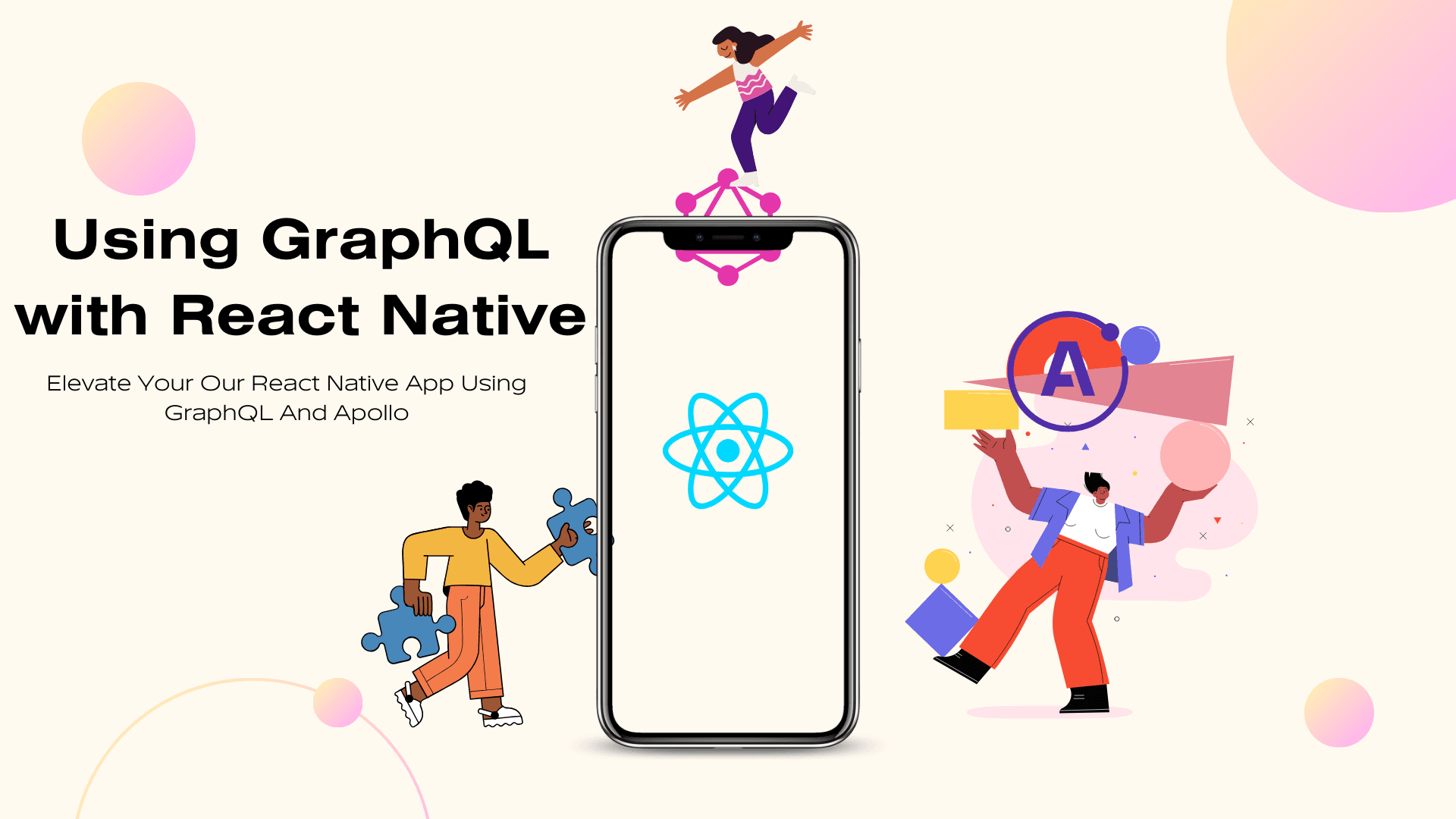
1. Introduction
In the realm of mobile development, achieving efficient data fetching is paramount for optimal app performance and user experience. GraphQL and React Native are two powerful technologies that, when combined, can significantly enhance data management and UI responsiveness. GraphQL is a query language for your API and a server-side runtime for executing queries using a type system defined for your data. React Native, on the other hand, is a framework for building native apps using React, allowing developers to write applications for iOS and Android using a single codebase.
2. Setting Up Apollo Client
To integrate GraphQL with React Native, one popular approach is using the Apollo Client. Apollo Client is a comprehensive state management library for JavaScript that enables you to manage both local and remote data with GraphQL.
To get started, install the necessary packages:
npm install @apollo/client graphql
Next, set up Apollo Client in your React Native application:
import { ApolloClient, InMemoryCache, ApolloProvider } from '@apollo/client';
const client = new ApolloClient({
uri: '<https://your-graphql-endpoint.com/graphql>',
cache: new InMemoryCache()
});
const App = () => (
<ApolloProvider client={client}>
<YourRootComponent />
</ApolloProvider>
);
This setup initializes Apollo Client with your GraphQL endpoint and integrates it into your React Native app using the ApolloProvider component.
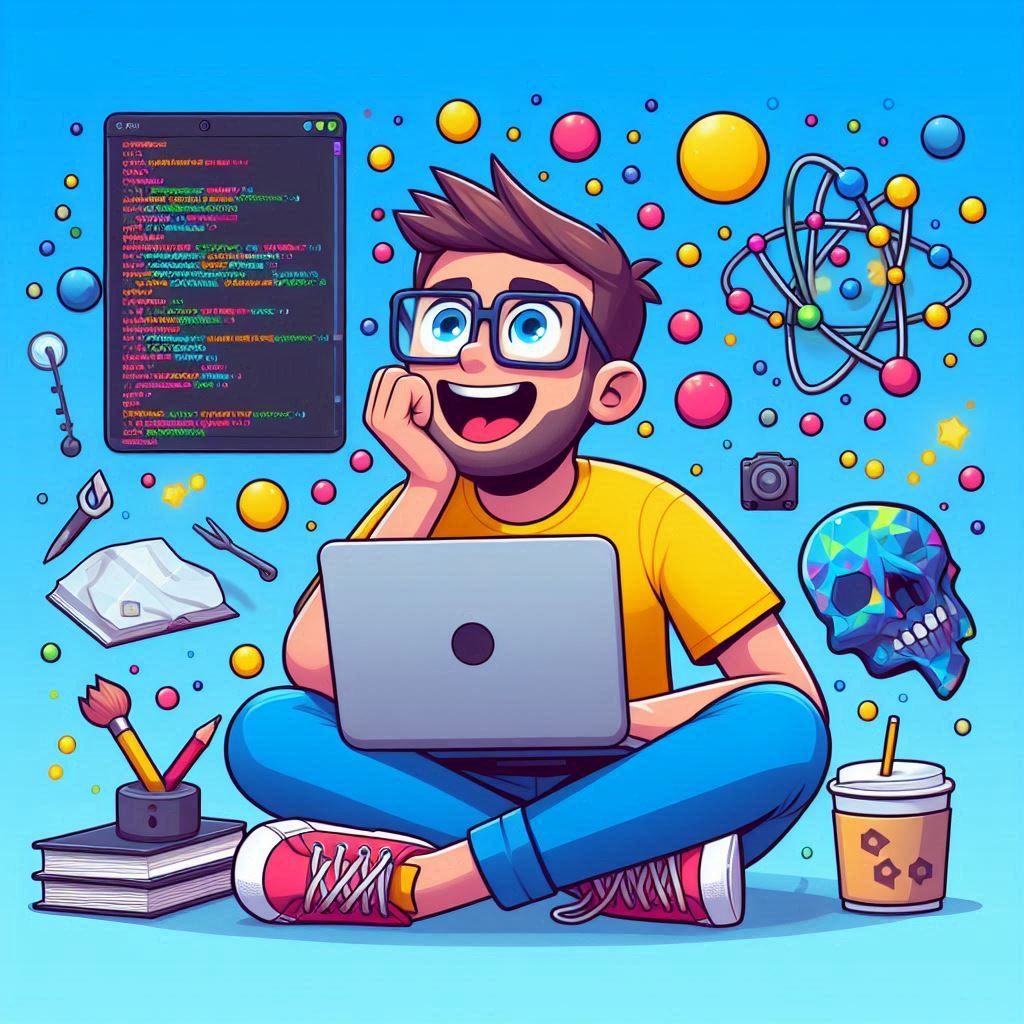
3. Writing Efficient GraphQL Queries
Writing efficient GraphQL queries is crucial for reducing load times and improving app responsiveness. Here are some best practices:
Fragment Usage: Reuse common fields across multiple queries by using fragments.
fragment UserDetails on User {
id
name
email
}
query GetUser($id: ID!) {
user(id: $id) {
...UserDetails
}
}
query GetUsers {
users {
...UserDetails
}
}
Pagination: Use cursor-based pagination to efficiently fetch large lists of data.
query GetPaginatedUsers($first: Int, $after: String) {
users(first: $first, after: $after) {
edges {
node {
id
name
email
}
}
pageInfo {
endCursor
hasNextPage
}
}
}
4. Handling Local State Management
Apollo Client also allows you to manage local state alongside remote data. Here's how you can define a local schema and resolvers:
const client = new ApolloClient({
uri: '<https://your-graphql-endpoint.com/graphql>',
cache: new InMemoryCache(),
resolvers: {
Mutation: {
updateLocalState: (_, { newState }, { cache }) => {
cache.writeData({ data: { localState: newState } });
return null;
}
}
}
});
This setup enables you to manage local state using the same GraphQL queries and mutations you use for remote data.
5. Best Practices for Optimizing Data Fetching
To optimize data fetching, consider the following best practices:
Batching Queries: Use Apollo Link to batch multiple queries into a single network request.
import { BatchHttpLink } from '@apollo/client/link/batch-http';
const httpLink = new BatchHttpLink({
uri: '<https://your-graphql-endpoint.com/graphql>',
batchInterval: 10
});
const client = new ApolloClient({
link: httpLink,
cache: new InMemoryCache()
});
Caching: Leverage Apollo Client's caching capabilities to reduce redundant network requests.
- Normalized Cache: Automatically normalizes and caches data based on your queries.
- Cache Updates: Manually update the cache after mutations to ensure UI consistency.
client.mutate({
mutation: UPDATE_USER,
variables: { id, name, email },
update: (cache, { data: { updateUser } }) => {
const data = cache.readQuery({ query: GET_USERS });
cache.writeQuery({
query: GET_USERS,
data: {
...data,
users: data.users.map(user =>
user.id === updateUser.id ? updateUser : user
)
}
});
}
});
6. Case Studie
Case Study: A leading e-commerce platform successfully implemented GraphQL in their React Native application. By switching from REST to GraphQL, they reduced data over-fetching and improved app performance. The outcome was a 30% improvement in load times, an enhanced user experience and streamlined state management.
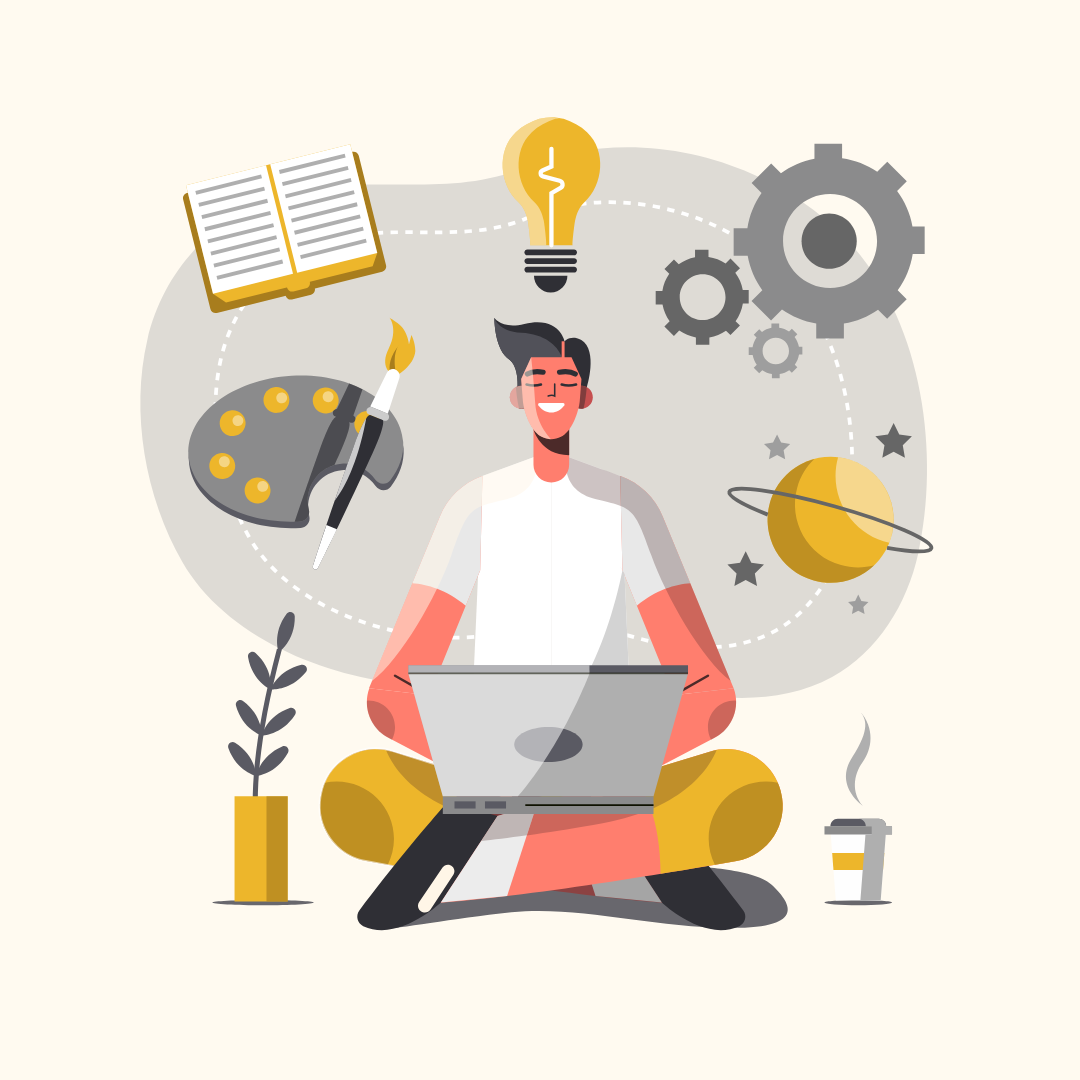
7. Conclusion
Combining GraphQL with React Native can significantly enhance the performance and maintainability of your mobile applications. By implementing best practices such as efficient querying, state management, and caching, developers can create more responsive and scalable applications. Leveraging the power of GraphQL and Apollo Client leads to optimal performance and an improved user experience, ensuring your app stands out in the competitive mobile landscape.
By following these guidelines, developers can ensure efficient data fetching in their React Native applications, fully utilizing the capabilities of GraphQL and Apollo Client for superior performance.
Until the next post happy coding fellas 😉.