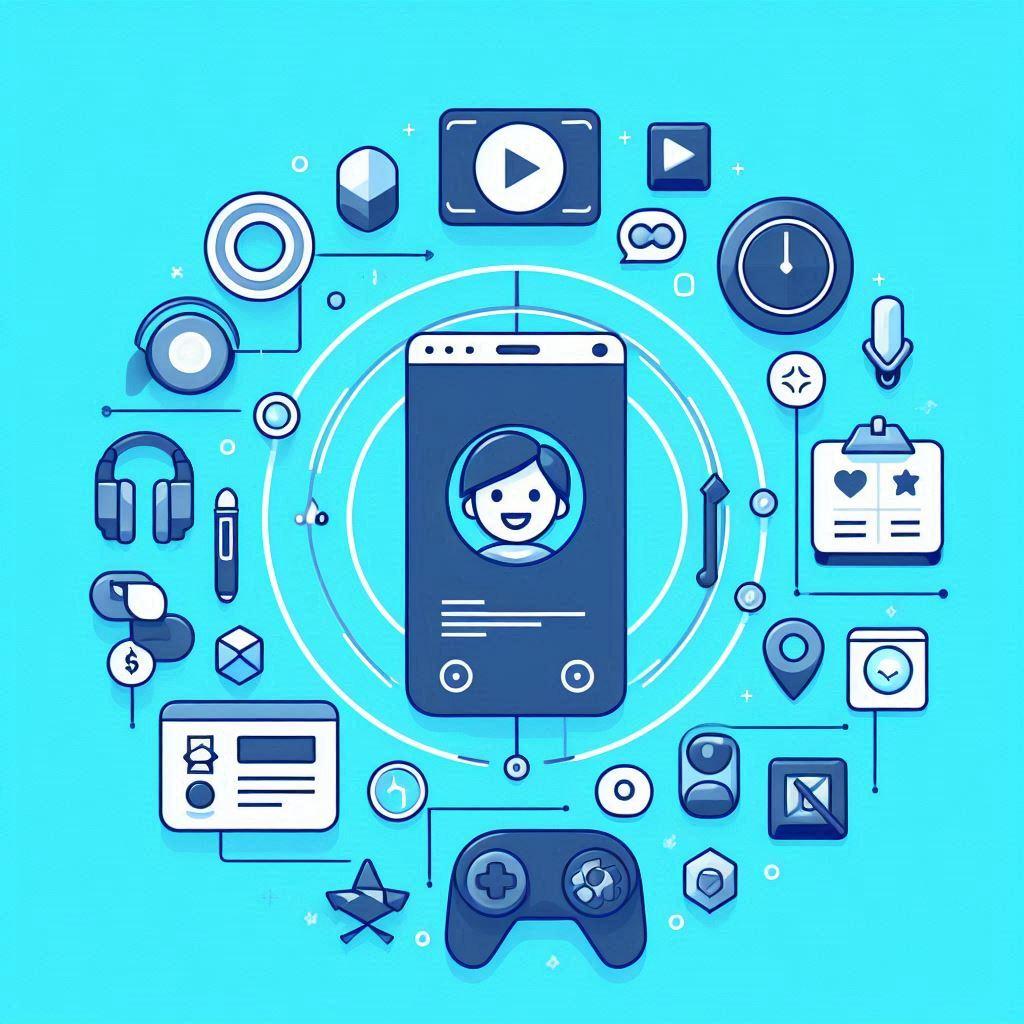
In the competitive world of SaaS applications, keeping users engaged isn’t just a goal—it’s a necessity. Enter gamification: the art of integrating game-like elements into your app to captivate users and keep them coming back for more. When applied to React Native, gamification can elevate your app’s user experience, enhance retention rates and make your mobile app truly stand out.
In this post, we’ll explore practical ways to incorporate gamification in React Native applications, share real-life examples and even dive into some code snippets. Let’s get started!
Why Gamification Works in SaaS
Gamification taps into basic human psychology by leveraging:
- Achievement: Users love completing tasks and earning rewards.
- Competition: A leaderboard can drive engagement by sparking a friendly rivalry.
- Exploration: Unlocking new features or levels keeps users curious and engaged.
- Recognition: Acknowledging user effort fosters loyalty and satisfaction.
- Belonging: Every improvement that will make users feel special and attract their attention will increase your users' sense of belonging.
By weaving these elements into your React Native app, you can transform mundane interactions into compelling experiences that boost customer retention.
Gamification Tricks for React Native Applications
Here are some tried-and-tested gamification features you can implement in your app:
1. Point Systems and Rewards
Encourage users to complete specific actions by awarding them points. For example, you can reward users for logging in daily, completing tutorials, or sharing your app with others.
Code Example
Here’s how you can implement a simple point system using React Native and AsyncStorage:
import React, { useState, useEffect } from 'react'; import { View, Text, Button } from 'react-native'; import AsyncStorage from '@react-native-async-storage/async-storage'; const RewardSystem = () => { const [points, setPoints] = useState(0); const fetchPoints = async () => { const storedPoints = await AsyncStorage.getItem('userPoints'); setPoints(storedPoints ? parseInt(storedPoints, 10) : 0); }; useEffect(() => { fetchPoints(); }, []); const addPoints = async () => { const newPoints = points + 10; setPoints(newPoints); await AsyncStorage.setItem('userPoints', newPoints.toString()); }; return ( <View> <Text>Your Points: {points}</Text> <Button title='Earn 10 Points' onPress={addPoints} /> </View> ); }; export default RewardSystem;
Use packages like react-native-confetti-cannon to make earning rewards visually exciting.
2. Leaderboards
A leaderboard fosters competition and encourages users to outperform their peers.
Real-Life Example
Consider fitness apps like Strava that use leaderboards to showcase the fastest runners or cyclists. Adding this feature to your app can motivate users to engage more frequently.
For leaderboard functionality, you can use Firebase Realtime Database:
import { getDatabase, ref, set, onValue } from 'firebase/database'; const db = getDatabase(); const leaderboardRef = ref(db, 'leaderboard'); const addScore = (userId, score) => { set(ref(db, `leaderboard/${userId}`), { score, }); }; const fetchLeaderboard = (setData) => { onValue(leaderboardRef, (snapshot) => { const data = snapshot.val(); setData(Object.entries(data).sort((a, b) => b[1].score - a[1].score)); }); };
Display the leaderboard in your app using a FlatList.
3. Achievement Badges
Reward users with badges when they achieve milestones. This taps into the desire for recognition and progress.
Tools to Use
- react-native-vector-icons: Create visually appealing badges.
- react-native-paper: A library for building beautiful badges and UI elements.
- Lottie Animations: Use animations to make the experience delightful.
- react-native-share: Allow users to share their achievements on social media.
Example Scenario
A productivity app could award badges for completing a task streak, such as “5 Days of Focus.”
import { Badge } from 'react-native-paper'; const AchievementBadge = () => ( <Badge style={{ backgroundColor: 'gold', color: 'black' }}>Streak 5 Days!</Badge> );
4. Progress Bars and Levels
Visual cues like progress bars and level indicators motivate users to keep going.
Real-Life Example
Apps like Duolingo show a progress bar for lessons, keeping learners engaged.
Here’s how to create a progress bar in React Native using react-native-elements:
import { ProgressBar } from 'react-native-elements'; const ProgressBarExample = ({ progress }) => ( <ProgressBar progress={progress} color='blue' /> ); export default ProgressBarExample;
Adding Levels
Combine progress bars with leveling-up animations using react-native-animatable:
import * as Animatable from 'react-native-animatable'; const LevelUp = ({ level }) => ( <Animatable.Text animation='bounceIn' style={{ fontSize: 24, color: 'green' }}> Level {level} Achieved! </Animatable.Text> );
Going A Step Forward And Dynamic Progress Visualization
Show users their progress in real-time using interactive charts or bars. Use react-native-chart-kit to visualize their achievements.
Example: Progress Tracker
import { ProgressChart } from 'react-native-chart-kit'; const data = { labels: ['Tasks', 'Challenges', 'Rewards'], data: [0.4, 0.6, 0.8], }; const ProgressVisualization = () => ( <ProgressChart data={data} width={320} height={220} chartConfig={{ backgroundGradientFrom: '#1E2923', backgroundGradientTo: '#08130D', color: (opacity = 1) => `rgba(26, 255, 146, ${opacity})`, }} /> ); export default ProgressVisualization;
5. Daily Challenges
Add daily tasks or challenges to encourage repeat visits. Use countdown timers to create a sense of urgency.
Packages to Use
- react-native-countdown-timer-hook: Easy-to-implement countdown functionality.
- redux-persist: Persist daily challenge states.
Enhanced Features
Integrate react-native-countdown-component to display countdown timers for challenges.
Visual Example
Create engaging banners with react-native-snap-carousel to showcase daily challenges.
import Carousel from 'react-native-snap-carousel'; const challenges = [ { title: 'Complete 3 tasks!', image: 'path/to/image1.png' }, { title: 'Log in for 7 days!', image: 'path/to/image2.png' }, ]; const ChallengeCarousel = () => ( <Carousel data={challenges} renderItem={({ item }) => ( <View> <Image source={{ uri: item.image }} /> <Text>{item.title}</Text> </View> )} sliderWidth={300} itemWidth={250} /> );
6. Mystery Boxes
Surprise users with random rewards through interactive mystery boxes. This keeps them curious and engaged.
Example: Mystery Reward
Use react-native-modal for a delightful reveal experience:
import Modal from 'react-native-modal'; import { Button, View, Text } from 'react-native'; const MysteryBox = ({ visible, onClose }) => ( <Modal isVisible={visible} onBackdropPress={onClose}> <View style={{ padding: 20, backgroundColor: 'white', borderRadius: 10 }}> <Text>🎉 You’ve unlocked a reward! 🎉</Text> <Button title='Claim' onPress={onClose} /> </View> </Modal> ); export default MysteryBox;
BONUS: React Native Walkthrough Onboarding & Highlighting Components
Implementing a walkthrough or onboarding experience in your React Native app can significantly improve user engagement and understanding. Here's how to create an effective walkthrough component:
import React from 'react'; import { View, Text } from 'react-native'; import Tooltip from 'react-native-walkthrough-tooltip'; const WalkthroughExample = () => { const [showTip, setShowTip] = useState(true); return ( <Tooltip isVisible={showTip} content={<Text>Welcome! This is your dashboard.</Text>} placement='bottom' onClose={() => setShowTip(false)} > <View style={styles.dashboard}> <Text>Dashboard Content</Text> </View> </Tooltip> ); };
Benefits of Walkthrough Components
- Improved User Onboarding: Guide new users through your app's features effectively, reducing confusion and frustration.
- Reduced Support Tickets: Well-designed walkthroughs can answer common questions before they arise, decreasing support workload.
- Higher Feature Adoption: Users are more likely to use advanced features when they understand how they work.
- Increased User Retention: A smooth onboarding experience leads to better user retention rates.
Best Practices for Implementation
When implementing walkthrough components, consider these key points:
- Keep it Progressive: Show information gradually rather than overwhelming users with everything at once.
- Make it Skippable: Always provide an option to skip the walkthrough for experienced users.
- Use Visual Cues: Implement highlights, tooltips, and arrows to draw attention to important features.
- Track Completion: Store walkthrough progress to avoid showing the same tips repeatedly.
// Example of tracking walkthrough progress import AsyncStorage from '@react-native-async-storage/async-storage'; const trackWalkthroughProgress = async (step) => { try { await AsyncStorage.setItem('walkthroughComplete', JSON.stringify({ completed: true, lastStep: step })); } catch (error) { console.error('Error saving walkthrough progress:', error); } };
Popular packages for implementing walkthroughs include:
- react-native-copilot: For step-by-step guided tours
- react-native-walkthrough-tooltip: For customizable tooltips
- react-native-onboarding-swiper: For swipeable onboarding screens
Remember to test your walkthrough with real users and iterate based on feedback to create the most effective onboarding experience possible.
Tips for Implementing Gamification
- Keep It Simple: Overcomplicating gamification elements can overwhelm users.
- Reward Meaningful Actions: Focus on behaviors that align with your app’s goals.
- Test and Iterate: Use A/B testing to identify what works best for your audience.
- Personalize the Experience: Dynamic content based on user preferences boosts engagement.
- Use Analytics: Monitor how users interact with gamified features using tools like Amplitude or Mixpanel.
Benefits of Gamification in SaaS
- Increased Engagement: Gamification transforms routine tasks into enjoyable experiences.
- Improved Retention: Features like streaks and challenges keep users coming back.
- Enhanced User Experience: Personalized elements make users feel valued.
- Boosted Motivation: Unlocks and rewards give users goals to strive for.
Final Thoughts
Gamification is more than just a trend; it’s a proven strategy to boost engagement and customer retention. By leveraging React Native’s flexibility and a rich ecosystem of third-party libraries, you can create delightful experiences that keep users hooked. Start small, test features and build upon what resonates with your audience.
Gamification can be achieved not only with the steps listed above, but also sometimes with small app-specific add-ons. And this can be done with some minimalist enhancements that impact the customer, such as an app-specific character that pops out of the side of the screen on the home screen to read the news of the day or notify them of the latest developments, or even an in-app, app-specific notification bar is an enhancement that makes the customer feel special.
Ready to gamify your app? Let us know your favorite gamification feature in the social media!