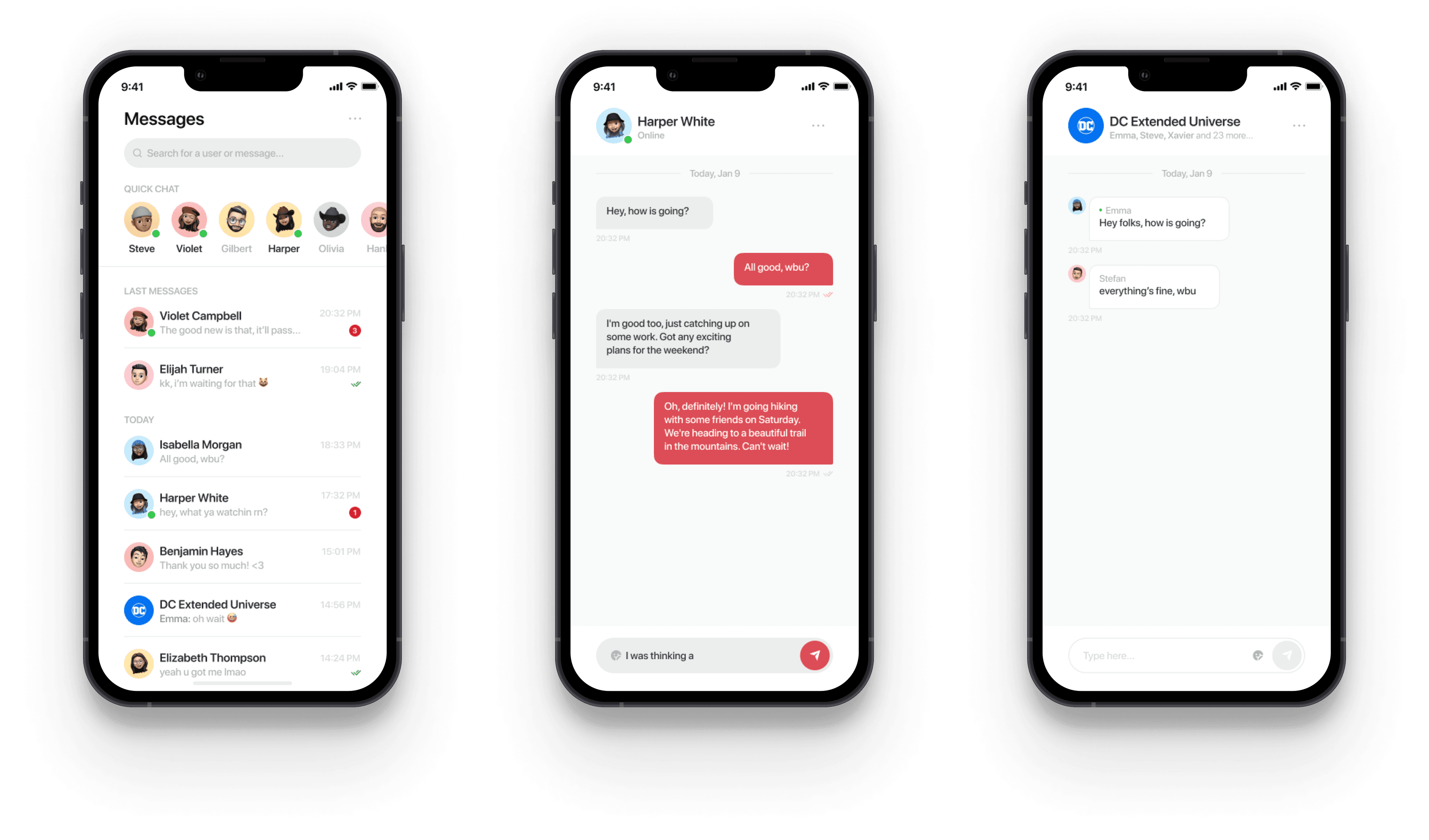
Welcome! In this tutorial, we'll guide you through the process of effortlessly building chat app with React Native by integrating Commt into, bringing a new level of realtime communication to your users.
Initializing Commt in Your App
To kickstart the integration, ensure you wrap your app with the CommtProvider and use the useInitiate hook to initialize the package. The tenant props, including the apiKey, subscriptionKey and tenantId, need to be provided to ensure a secure and personalized connection.
// App.tsx
import CommtProvider from "@commt/rn-sdk";
import { useInitiate } from "@commt/rn-sdk/hooks";
const TenantProps = {
apiKey: "123456789?",
subscriptionKey: "0987654321?",
tenantId: "AD768342asdf?9892",
};
function App(): JSX.Element {
useInitiate(TenantProps);
return (
<CommtProvider>
{/* All your ThemeProvider, NavigationContainer, etc. */}
</CommtProvider>
);
}
Setting Up Commt Data
To ensure the smooth functioning of Commt's pre-built components, make sure to set up crucial data, including users list, rooms list and messages, in a strict format with the correct priority (users -> rooms -> messages).
// Home.tsx
import { useSetMessages, useSetRooms, useSetUsers } from "@commt/rn-sdk/hooks";
const Home = () => {
// ...
const isUsersSet = useSetUsers(usersArray);
const isRoomsSet = isUsersSet && useSetRooms(roomsArray);
isUsersSet && isRoomsSet && useSetMessages(messagesArray);
// ...
return (
<SafeAreaView>
<Text
style={styles.buttonText}
onPress={() => navigation.navigate("Messages")}>
Go Messages
</Text>
</SafeAreaView>
);
};
Pre-Built Chat Components
In your messages list screen, you can import and use pre-built react native chat components such as MessageList, QuickChat.
// MessageList.tsx
import {
QuickChat,
MessageList,
SearchInput,
MessagesHeader
} from "@commt/rn-sdk/components";
const Messages = () => {
// ...
return (
<Container>
<MessagesHeader />
<SearchInput />
<InnerContainer>
<QuickChat onPress={navigateToChat} />
<MessageList onPress={navigateToChat} />
</InnerContainer>
</Container>
);
};
With Commt seamlessly integrated, you can effortlessly add pre-built chat components to your app. In your chat screen, use the ChatHeader and Chat components, passing the required parameters for them to function correctly.
// Chat.tsx
import { ChatHeader, Chat } from "@commt/rn-sdk/components";
const Chats = () => {
const route = useRoute<RouteProp<RootStackParamList, "Chats">>();
const roomId = route.params.roomId;
const participants = route.params.participants;
return (
<Container>
<ChatHeader roomId={roomId} participants={participants} />
<Chat roomId={roomId} participants={participants} />
</Container>
);
};
Conclusion
With Commt's pre-built chat components and hooks, you can seamlessly integrate real-time chat into your app. Stay tuned for our next post, where we'll delve into the world of security, exploring how to implement end-to-end encryption with Commt and build realtime secure chat app. See you there!